Some of Storage services are not free like amazon s3 or cloud, and I was also wanted to get free storage, I found #Dropbox which is providing 5GB free space.
Before starting this tutorial you must have following requirements.
Requirements
PHP version 7.1 or 7.2 or 7.+
Laravel latest framework
benjamincrozat/laravel-dropbox-driver package
Create/Install Laravel
Let’s create/install Latest Laravel through #CLI with following command in your project directory.
composer create-project --prefer-dist laravel/laravel laraveldropbox
After the installation of Laravel Framework install
Install Laravel Dropbox Driver Package
Here we will through #CLI install package to communicate dropbox. Open command/cli in your installed Laravel directory and run following command
composer require benjamincrozat/laravel-dropbox-driver
Create app and generate access token
Register account or login to to create app and generate dropbox access token from following url https://www.dropbox.com/developers/apps
Fill the following fields and click on create app button.

click to create dropbox app
Generate Dropbox App access token
Go to your app and click on generate for generating access token. keep that token in your file we will define in .env file in next step.
dropbox-generate-access-token-button
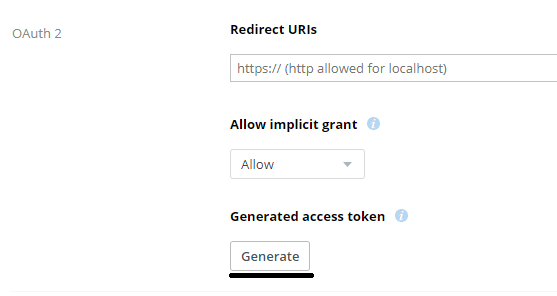
dropbox-generate-access-token-button
Define token in .env file
DROPBOX_TOKEN=PASTE_HERE_GENERATED_TOKEN
Integrate /Configure benjamincrozat/laravel-dropbox-driver
Including providers in config/app .file
'providers' => [ BC\Laravel\DropboxDriver\ServiceProvider::class, ],
Adding Following Dropbox array to Disk configs in config/filesystem.php
'dropbox' => [ 'driver' => 'dropbox', 'token' => env('DROPBOX_TOKEN'), ],
Create Controller
Create Controller file DropboxController.php in app/Http/Controllers directory with following code
namespace App\Http\Controllers; use Illuminate\Routing\Controller as BaseController; use Illuminate\Support\Facades\View; class DropboxController extends BaseController{ public function uploadToDropbox(){ return View::make('dropbox'); } }
Define route for uploading
Write following lines in web.php file for route to upload file on Dropbox
Route::get('/upload-to-dropbox','DropboxController@uploadToDropbox');
Create view
Let’s create view file in resources\views directory and named it “dropbox.blade.php” here we will use #bladeTemplate
Write following code in file
<!doctype html> <html lang="{{ app()->getLocale() }}"> <head> <meta charset="utf-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1"> <title>Upload to Dropbox</title> <link href="https://fonts.googleapis.com/css?family=Raleway:100,600" rel="stylesheet" type="text/css"> <!-- Styles --> <style> html, body { background-color: #fff; color: #636b6f; font-family: 'Raleway', sans-serif; font-weight: 100; height: 100vh; margin: 0; } .full-height { height: 100vh; } .flex-center { align-items: center; display: flex; justify-content: center; } .title { font-size: 84px; } .m-b-md { margin-bottom: 30px; } </style> </head> <body> <div class="flex-center position-ref full-height"> <div class="content"> @if($errors->any()) <h4>{{$errors->first()}}</h4> @endif <div class="title m-b-md"> Upload file to dropbox <form enctype="multipart/form-data" method="post" action="upload_to_dropbox"> <input type="hidden" value="{{csrf_token()}}" name="_token" /> <input type="file" name="upload_file" /> <input type="submit" value="Upload to Dropbox" /> </form> </div> </div> </div> </body> </html>
Access it on your local host with following url
localhost/laraveldropbox/public/upload-to-dropbox/
laravel remove public from url
Create route to hand/upload file
Write following line into your routes/web.php routing file for handling when file uploaded and sent to your server.
Route::post('/upload_to_dropbox','DropboxController@uploadToDropboxFile');
Add Name Spaces in DropboxController file
namespace App\Http\Controllers; use Illuminate\Routing\Controller as BaseController; use Illuminate\Support\Facades\View; use Illuminate\Http\Request; use Illuminate\Support\Facades\Storage; use Illuminate\Support\Facades\Redirect;
Create Method for handling and uploading file
Create Method
public function uploadToDropboxFile(Request $RequestInput){ $file_src=$RequestInput->file("upload_file"); //file src $is_file_uploaded = Storage::disk('dropbox')->put('public-uploads',$file_src); if($is_file_uploaded){ return Redirect::back()->withErrors(['msg'=>'Succesfuly file uploaded to dropbox']); } else { return Redirect::back()->withErrors(['msg'=>'file failed to uploaded on dropbox']); } }
Note: for learn more methods for file system eg: move file to another location, delete or modify click on following link https://laravel.com/docs/5.6/filesystem
-
Hello,
I been able to upload file to Dropbox using your tutorial.
How to get the uploaded file’s download-able URL?
Thanks.